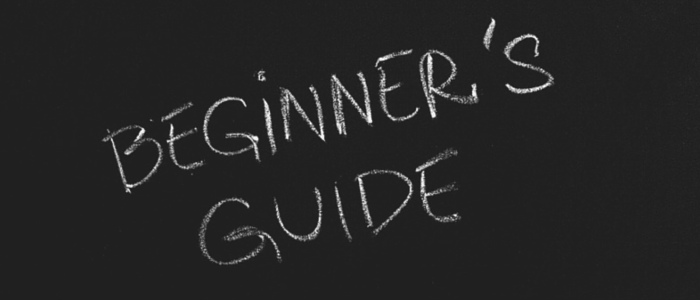
A Beginners Guide for WordPress Themes Development
This post is aimed at beginners, it does presume you’ve tinkered with WordPress before and you know basic HTML. I will assume that you know how to edit WordPress files and you’ve looked into a WordPress theme file – even if you didn’t understand what’s going on.
The Programming Languages Of WordPress
WordPress uses a number of different programming languages. If one language has to be singled out as the “main” one it would be PHP. PHP is a server side language, which powers about 82 percent of the web.
WordPress also uses HTML, CSS and Javascript. HTML is used to give your website structure and is employed by all websites. CSS helps style your HTML structure. CSS makes your background white, your text dark-grey and positions the sidebar on the right. Javascript adds advanced features like sliders and other interactive features.
Finally, WordPress also uses MySQL, which is responsible for querying the database. MySQL is used to retrieve the last 10 posts, or all posts in a particular category from the database.
OK, so the bad news is that this is a considerable body of knowledge. The good news is that you don’t need to know everything to get started; in fact, you can get by with very little.
What WordPress is Not
It is important to realize that technically there is no such thing as “WordPress coding” and “WordPress code.” WordPress is a bunch of code written in PHP. Joomla and Drupal (two other content management systems) are also written in PHP.
Analogy to the rescue! Saying “WordPress code” is like saying “BMW Car.” A BMW, a Jaguar and a Nissan are all cars – they are all built with nuts, bolts and welding. The difference between them is how they are put together, the design philosophies and the assemblage practices.
WordPress, Joomla, Drupal and all the other systems and frameworks out there are all built with the same components. The difference between them is the coding philosophy and methodologies they employ.
How PHP Works
As I mentioned earlier, PHP is a server-side scripting language. In contrast, HTML is a client-side language. Let’s analyze HTML first to understand what this means.
The way your browser interprets HTML code is the following: When you visit an HTML page the HTML code is sent to your browser. Your browser processes the information and spits out something you recognize as a web page.
When your browser visits a page that employs PHP, an intermediate step is employed. First the PHP code is processed by the server. The result of this processing is an HTML page, which is then sent to your browser and displayed to you.
The additional processing by the server seems like an unnecessary step but far from it. Let’s look at a practical example with real PHP code:
<?php if( date( 'G' ) > 18 ) : ?> <h2>Good Night!</h2> <?php else : ?> <h2>Good Day </h2> <?php endif ?>
Without any understanding of PHP code, we can already gather some information about it. Just by reading it you can discern that if a particular set of circumstances is true we display “Good night,” otherwise we display “good day.”
When you look at the source of the resulting web page there will be no trace of this code. All you will see is either “Good day” or “Good night.” This is because the server does the processing and only sends you the result.
PHP in WordPress
With that last paragraph in mind, you can recognize PHP everywhere in WordPress. Let’s open the content.php
from the Twenty Fourteen default theme and take a look. This file is responsible for displaying the content of blog posts in the theme.
Let’s compare the first line of this file (discarding the comment on top)…
<article id="post-<?php the_ID(); ?>" <?php post_class(); ?>>
…with the output it generates when you visit the page:
<article id="post-344" class="post-344 post type-post status-publish format-standard has-post-thumbnail sticky hentry category-photos tag-example tag-tag">
We can deduce from the comparison that the the_ID()
function is replaced by the ID of the post in question. The post_class()
function adds a lot of classes to the HTML element. These help us in styling our posts later on. It’s not important at this stage to know why these specific classes are added, we’re just familiarizing ourselves with functions.
Further on, looking at lines 24 through 28 we can also see an if statement at work:
if ( is_single() ) : the_title( '<h1 class="entry-title">', '</h1>' ); else : the_title( '<h1 class="entry-title"><a href="' . esc_url( get_permalink() ) . '" rel="bookmark">', '</a></h1>' ); endif;
The if statement has is_single()
in it. This is a function which will be true if we are looking at a single post page, otherwise it will be false. If it is true and we are on a single page, we use the the_title()
function to output the title.
If it is false, we still use the the_title()
function, but we make sure it is a link to the single post page.
Notice that some functions are “empty” while some have bits and pieces within them. For example, is_single()
is an empty function while the_title()
has some gunk within the parenthesis.
The items within the parenthesis are called arguments. Each function has different arguments separated by commas, which you can learn about through documentation. The Codex article on the_title() shows us that this function has three arguments:
- The first argument allows us to add HTML before the title,
- The second allows us to add HTML after the title, and
- The third parameter determines weather the title is shown (echoed) or it is just stored for use later.
Based on this, we now understand what’s going on in line 25 of the content.php
file:
the_title( '<h1 class="entry-title">', '</h1>' );
The function shows the title but we prepend an H1 starting tag to it and append the end tag.
The result of this code looks like this in the browser:
<h1 class="entry-title">THe title of my article</h1>
How to Level Up in WordPress Programming
Chances are you don’t want to spend weeks wading through PHP documentation and learning about everything from the ground up. You should do this, but I also recommend you experiment as much as possible.
Want to move the list of tags from the bottom of the article to the top? Thethe_tags()
function at the bottom of the content.php
file looks promising.
First, let’s delete it together. Then, when you save and refresh the page, the tag list if gone. This is great, it means that this is indeed the function that outputs the tags. Now just copy it and paste it in to various parts of the file to see where it ends up.
it is probable that the higher up you go in the code, the higher up it will be in the article. With some experience you’ll be able to identify things like the_excerpt()
and the_content()
being responsible for displaying the content, so putting it anywhere above those will place it above the main content.
Learning how to code for WordPress this way is fun and encourages you to read the documentation, which is always a good thing. Don’t worry if you don’t understand everything – you will reach a point when you do soon enough.
Learning Bad Practices
One drawback of this method is that you will employ bad practices. While my recommendation that you copy-paste the the_tags()
function to the top of the file somewhere works, the HTML for the footer, which uses the footer tag, will need some modification to make it good code.
Again, forget about this for now. You are not building professional-grade production-ready code for Google. You are trying to learn the basics and figure out how everything works. This is not an easy task and mistakes are part of the process.
Once you have a good working knowledge of the code behind WordPress, you can start un-learning your bad practices and you can start studying coding patterns and figuring out why we do things the way we do.